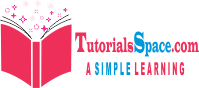
Built In And User Defined Data Structures
Built in or Primitive Data Structure :
Primitive data structures although data structures which cannot be further divided
Example:
A Date which is composed of DD,MM,YY so it cannot be Primitive Data Types.
Integer, Real(Float), boolean and characters
They are our "Builtin and Primitive Data Types".
Operation which can be applied on them:
Int= addition, abstraction, and other mathematical operation
+ , - , * , / , % etc.
Elementary Data Structure:
variable is the most fundamental Data Structure available to a programmer.
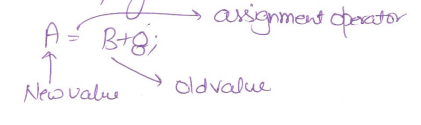
Elementary_Data_Structure
It can be used as a basic building block to construct more Complex data structure.
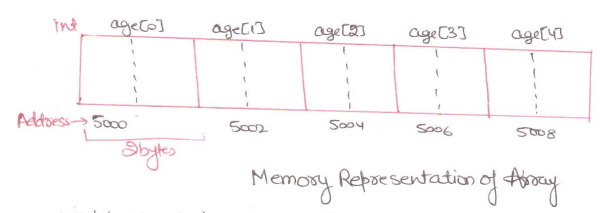
Initialization of 1 - dimensional array:
int age[5]={2,5,34,3,4};
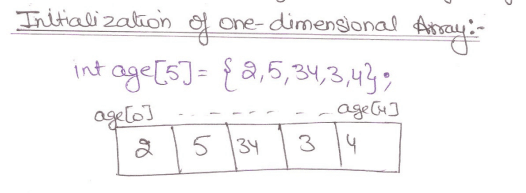
Program to find the total Marks of Many Students using Array in C
#include<stdio.h> void main() { int marks[10],i,n,sum=0; printf("Enter the number of students:"); scanf("%d", &n); for(i=0;i<n;i++) { printf("Enter the marks of students %d:",i++); scanf("%d,&marks[i]"); sum=sum+marks[i]; } printf("sum=%d",sum); } getch(); }
Output
Enter the no. of students:3 Enter the marks of student1:15 Enter the marks of student2 :20 Enter the marks of student3:10 sum=45
Arrays:
In C language if user wants to know marks of 100 students so he has to declare hundred variable individually but this process is not tedious and impracticable. This kind of problem can be handled in C with arrays.
Array is a collection of data of same type of variable which is referenced by a common name.
Arrays are of two types:
• One Dimensional Arrays
• Multi Dimensional Arrays
Declaration Of One Dimensional Array:
data_type array_name[array_size];
For example int age[5];
In this Name of the array is age and size of the array is 5 i.e there are 5 items (element) a array age and all elements in an array are of same size and same type.
Array elements:
Size of Array defines the number of elements in an array and each element can be accessed according to requirement.
int age[5]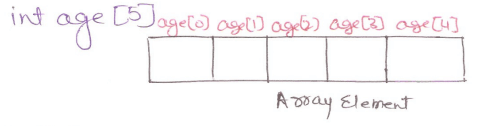
Not that the first element is number 0 and so on.
03- BuiltIn and User Defined Data Structures Part-1- DataStructure
CLICK HERE TO Download This PDF NOTES