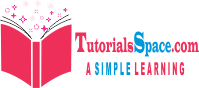
Structures
As we saw arrays contains elements of Same data types. But if we need something with contains element of different data type. So structure basically helps us in this.
It is a collection of different data types which referred under one name.
Like information about a student composed of many different data types like name, age, gender, roll number, class.
Structure definition of structure designing and variables:
We use keyword struct followed by its name and body enclosed in curly bracket. Body of the structure contains the definition of its members and each member must have name.
declaration ends up by ; and after that structure variables are declared.
struct{ member1; member2; . . . member n; } ; structure variable name
Example
Struct student { char name[20]; int age; int roll; char class[10]; } ; stud1, stud2
How To Accessing Structure Elements:
There are two ways of accessing structure elements
• 1) .Dot Operator or Structure Member Operator: It accesses a structure member via structure variable
Example stud1.age;
• 2) Structure Pointer Operator(->) or Arrow Operator: Suppose a structure member declared as int *gender // 1 for male and 2 for female And structure variable *stud3
so * stud3 a pointer which has been declared to point to struct student and the address of structure *gender has been assigned to stud3. So to print it, we will use stud3->gender
Memory allocation of structure:
As soon as we declared structure name and the body element with its variable space allocated to the memory.
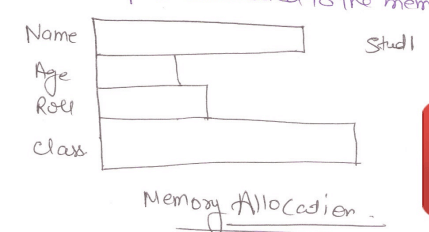
/* simple and complete program for structure*/ #include#include oid main() { struct student { char name[20]; int age; int roll, sub1,sub2,sub3,sub4; int total; }; student stud; // variable declaration clrscr(); printf("enter the record of the student:"); printf("Name:"); scanf("%s\n",stud.name); printf("Age:"); scanf("%d\n",stud.age); printf("Roll no."); scanf("%d\n",stud.roll); printf(" Enter Marks of subject 1,2,3,4:"); scanf("%d%d%d%d\n", stud.sub1, stud.sub2,stud.sub3,stud.sub4); printf("grade of student %s is:", stud.name); stud.total=stud.sub1+stud.sub2+stud.sub3+stud.sub4; if(stud.total<240) printf("D"); else if(stud.total<320) printf("\B"); else print("A");
Arrays of structure:-
An array of structure can be declared as an ordinary array ans in same manner we will access the members of structure as we do by ordinary variable.
/* program to print 10 roll number*/ void main() { struct student { int roll; } stud[10]; printf(" enter 10 roll number"); for(int i=0;i<10;i++) { scanf("%d", stud[i].roll); } printf("The ten roll no. are "); for(i=0;i<10;i++) printf("%d/n", stud[i].roll); getch(); } output enter 10 roll no. 2 8 19 18 7 14 5 6 29 10 the 10 roll no. are 2 8 19 18 7 14 5 6 29 10
Explanation:
In this we declared structure variable stud[10] of student type.
We access the value same as we access with ordinary variables.
04- BuiltIn and User Defined Data Structures Part-2- DataStructure
CLICK HERE TO Download This PDF NOTES